How to use multiple post types in a Query Loop Block
Currently the Query Loop only supports one post type. In this article we will show how we add multiple post types to the blocks query using the pre_get_posts action.
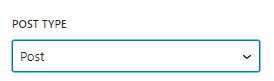
Share
To filter multiple post types in one Query loop block in WordPress, you need to customize your query using the pre_get_posts
action. To target a specific Query Loop block, which is probably what you want, you need to add a custom attribute or class to the Query Loop block in the Block Editor and then use that attribute or class in the pre_get_posts
function to conditionally modify the query.
Here is a step-by-step guide:
Step 1: Add a Custom Class to the Query Loop Block
- Open the page or post where you want to add the Query Loop block in the Block Editor.
- Add or select the Query Loop block.
- In the block settings on the right-hand side, expand the “Advanced” panel.
- Add a custom class name, for example, custom-query-loop, to the “Additional CSS class(es)” field.
Step 2: Use ‘pre_get_posts’ to Target the Custom Class
In your theme’s functions.php
file, add code to conditionally modify the query based on the presence of the custom class.
function filter_specific_query_loop($query) {
// Check if this is an admin query or not the main query
if (is_admin() || !$query->is_main_query()) {
return;
}
// Check for the presence of the custom query parameter
if (isset($query->query_vars['custom_query_loop']) && $query->query_vars['custom_query_loop']) {
// Modify the query to include multiple post types
$query->set('post_type', array('post', 'page', 'your_custom_post_type'));
}
}
add_action('pre_get_posts', 'filter_specific_query_loop');
// Add a filter to include the custom query parameter when rendering the block
function add_custom_query_var($block_content, $block) {
if ($block['blockName'] === 'core/query') {
if (isset($block['attrs']['className']) && strpos($block['attrs']['className'], 'custom-query-loop') !== false) {
add_filter('query_vars', function ($query_vars) {
$query_vars[] = 'custom_query_loop';
return $query_vars;
});
}
}
return $block_content;
}
add_filter('render_block', 'add_custom_query_var', 10, 2);
Explanation
- Filter the Query in
pre_get_posts
:- The
filter_specific_query_loop
function checks if the query is not for the admin area and if it is the main query. - It then checks for a custom query variable
custom_query_loop
. If this variable is set, it modifies the query to include multiple post types.
- The
- Add Custom Query Variable:
- The
add_custom_query_var
function checks if the block being rendered is a Query Loop block and if it has the custom classcustom-query-loop
. - If these conditions are met, it adds a custom query variable
custom_query_loop
to the query.
- The
Step 3: Ensure Query Loop Block Renders Correctly
Make sure your theme supports the query parameters correctly and that the pre_get_posts
hook properly modifies the main query. This approach ensures that only the specific Query Loop block with the custom class custom-query-loop
is modified to include multiple post types.
Additional Note
This method relies on adding a custom class and hooking into WordPress’s query system. If your setup or requirements are different, you may need to adjust the logic accordingly. Ensure that you have backup copies of your files before making changes to your theme.
Want to add filter and search to your Query Loop Block too?
Read our tutorial on using the Filter Query Block Pro plugin to easily add filters and search to your Query Loop block!
More tutorials
Top 10 Must Have Code Snippets to Supercharge Your WordPress Site
Ten essential code snippets that you can add to your functions.php file to enhance your WordPress site
Read more Top 10 Must Have Code Snippets to Supercharge Your WordPress Site
Understanding the WordPress Query Loop Block: What It Is and How to Use It
The Query Loop Block is a powerful feature in WordPress that allows users to display lists of posts or pages dynamically.
Read more Understanding the WordPress Query Loop Block: What It Is and How to Use It
How to add filters and search to the WordPress Query Loop Block
It has been a pain to add filters and search to your feeds in Gutenberg. Not any more, we will teach you…
Read more How to add filters and search to the WordPress Query Loop Block